Javascript User Defined Functions
The goal of this assignment is to practice your JS skills, as well as put together HTML, CSS, and JS in one page. Specifically, you will get practice with:
- reading from input elements on the web page
- writing on the web page and on the console
- defining functions with and without parameters
- adding of a responsive button on a web page
- use the click event to trigger the call of a function
Forming teams
You may work in pairs to complete this assignment, or you may work alone.
If you want to work with a partner, use this team-forming shared document to form and declare teams.
Set up and preparation
From your download directory, get the folder named
hw05
. It contains materials you will use for this
assignment. Open the .html and .js files provided to examine the code,
in your editor. Load the html documents on your browser, and see how
they look.
Note: For completing this assignment, you do not need to, and you should not edit the provided html documents. Write your js code in the js files. You will need to look at the html code to identify the IDs of a couple of elements to use in your js code.
Task 1: The Old MacDonald song
Remember this old children's song? Here is a verse of it:Old MACDONALD had a farm E-I-E-I-O And on his farm he had a cow E-I-E-I-O With a moo moo here And a moo moo there Here a moo, there a moo Everywhere a moo moo Old MacDonald had a farm E-I-E-I-OThere is plenty of repetition from verse to verse in the song. As a matter of fact, the only words that change from verse to verse are the animal and the sound it makes!
For this task, you will write a JavaScript program to dynamically write on a web page a few verses of this song. The fact that there is so much repetition from verse to verse, makes it an ideal case for the use of functions!
Open the oldMac.html
and create a new file oldMac.js
in your code editor (Atom), and open oldMac.html
in your
browser.
Examine the "oldMac.html" carefully, to understand the structure and names of
the elements on the page.
Task 1A: produceVerse()
function
Define the function produceVerse(animal,sound)
, in the
"oldMac.js" file, to return a string representing of an entire verse of the
Old MacDonald song, based on the inputs
animal
and sound
.
Invoke produceVerse()
from the console, using fixed values for the
arguments.
As an example, type at the console's prompt:
produceVerse("dog","woof");
You should see the string representing the HTML for the verse displayed in the console. If not, make sure to fix your program at this stage, before continuing.
Task 1B: updatePage()
function
Define a second function, named updatePage()
, which:
-
reads
the animal, and the sound the user enters in the two input boxes on the web page (oldMac.html
) -
calls the produceVerse()
function to produce the corresponding verse of the song, and... -
adds
that verse at the bottom of the page (oldMac.html
), after any verses that might be there already.
Click on the "Add Verse" button to test your work.
Add several verses to the song, and verify that the song is displayed on the page correctly. You should be able to see several verses of the song at the page, not only one.
Reading values from inputs
As a reminder here is how you can read the value from a text input with id "input1Id":
document.getElementById("input1Id").value;
Appending HTML
We've used.innerHTML =
to replace the contents of an element on
the page, but in order to append to an element instead of replacing its
content, you can use .innerHTML +=
instead.
Task 2: Meme Generator
For this task, you will write a meme generator, which will allow the user to create a custom meme (i.e., an image with some text on it). Here is an example of what it should look like (right-click and select "Open image in new tab" to view details):
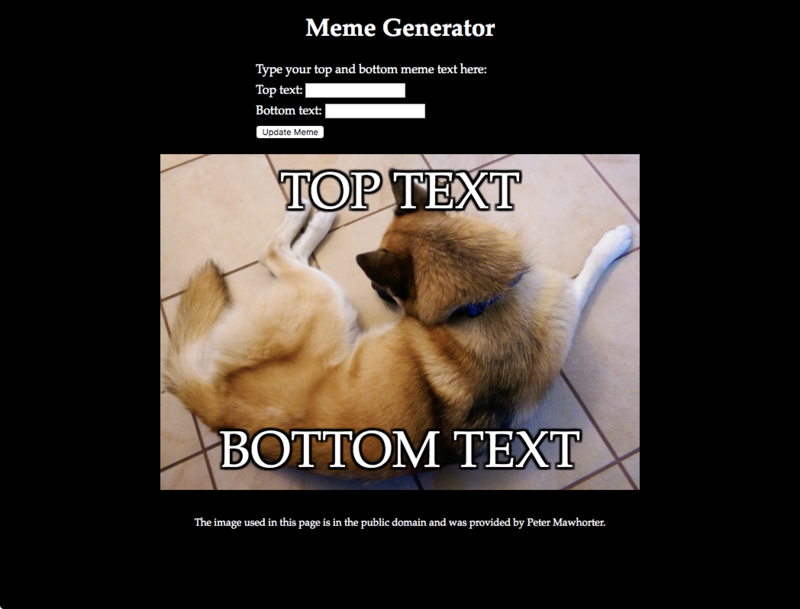
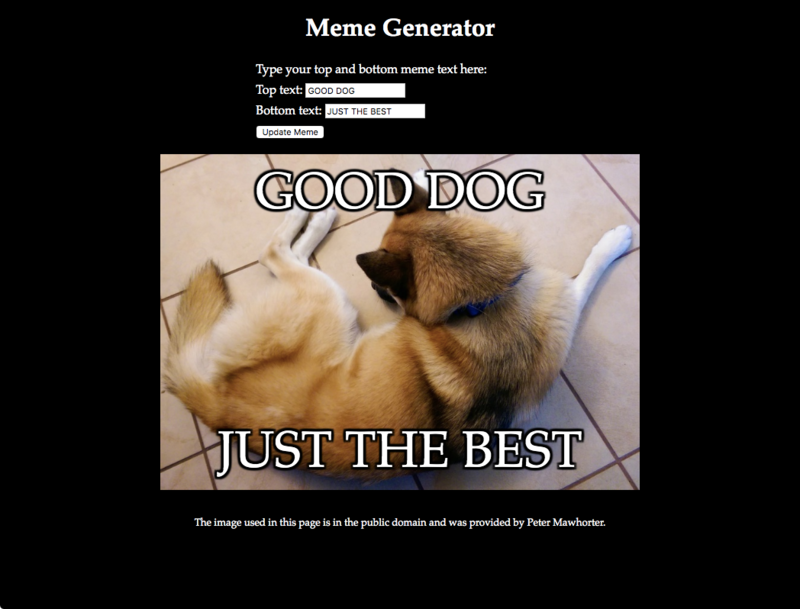
Here's another example of a meme using a custom image; this shows only the meme part:
Look at the provided file memes.html
, and see which js and CSS
files it links to. Also note which elements have ID values and what those IDs
are. In particular, we want to be able to change the value of the top and
bottom meme text, and we want to do that by using the values of the text
inputs.
For this task, you will be writing two JavaScript functions: one to handle updating the text, and another function to change the font size based on how much text there is.
Note: if you want, you may find a different image to use for your meme generator and update the HTML page to use that image. However, in that case you must also update both the alt text and the attribution text at the bottom of the page to accurately reflect how you are using the image. You should credit the original creator of the image if you can, and if it is a copyrighted image, you should state that you are using it under "fair use." Do not use an image counter to its creator's wishes.
Task 2A: updateMemeText()
function
You need to create the memes.js
file, and write your code in it.
To start with write the function updateMemeText()
, which does not
accept any arguments. This function should read the text values from the text
inputs on the page, and update the innerHTML
of the top and bottom
text spans to match the input values, similar to what you did in the previous
task. Once this is done, you should be able to click the "Update Meme" button
and see the meme text change.
Remember that if things aren't working, you should check the console for any error messages.
Task 2B: fontSizeForText()
function
Now that the meme generator is working, we'd like to do one more thing: change the font size depending on how much text the user enters. That way, even if they type a lot of text, it will still be able to fit on the image. To test this, try copying this paragraph of text and using it as the top text for the meme. You'll see that it is much too long, and runs over the whole picture and further down the page.
Your job is to build a function fontSizeForText(text)
. This
function will accept one argument, which should be a piece of text (a string). It will measure the length of the text, using:
var len = text.length;and then it will do the following:
- If the length is 40 or less, it should return the number 50, which is the default font size (it should return a number, not a string).
-
If the length is between 40 and 100 characters, it should return a number
between 50 and 30, depending on the length of the string.
Specifically, the closer to 100 characters in the text, the smaller the result should be. You can use a mathematical formula to compute how close to 40 or 100 the length is (as a number between 0 and 1), and then another formula to convert this number into a font size. Make sure to useMath.floor
at the end so that your result is an integer (and test this in the console). - If the text length is more than 100, it should return 30 (the minimum font size).
This text is exactly 30 chars. This text is exactly 40 characters long. Fifty characters is 1/6 of the way from 40 to 100. This text is 70 characters long, which is half-way between 40 and 100. This text is exactly 97 characters long, which is long enough to be almost the minimum font size. This text is exactly 116 characters long, which is long enough that it should definitely have the minimum font size.
Once you are done with your fontSizeForText
function and you have
tested it, you must modify your updateMemeText
function so that it
uses fontSizeForText
to compute the font size for the top and
bottom texts separately, and then in addition to updating the text of each
<span>
, it should update their font size as well.
Note that when updating font size, you can use
element.style.fontSize = number + "pt"
if you have an HTML element
to update stored in a variable element
, and a numerical font size
stored in a variable number
. This won't always work if the number
is not an integer though!
Bonus Task (no credit)
If you feel comfortable with the JavaScript code so far and want to go further on your own, try to add a drop-down selector to the page so that the user can choose from one of several images to use. Make sure you include attributions for all images if you do this.Submission
Your folder should contain the following files:
oldMac.html
oldMac.js
memes.html
memes.js
memes.css
dijkstra.jpg
Important Note
Please remember that if you work with a partner, both partners must submit the assignment to their accounts. Upload your files to your hw05 folder, inside thepublic_html/cs115-assignments
folder on your cs account.
Permissions have been set so that only you and the instructors/graders can
see your files there.
Finally, after you have uploaded your work, use the following URLs to access your files and make sure they work approrriately when accessed from the server.
http://cs.wellesley.edu/~yourname/cs115-assignments/hw05/oldMac.htmlWhen prompted for login credentials, enter your user name and password for your cs account.
http://cs.wellesley.edu/~yourname/cs115-assignments/hw05/memes.html
Due Date/Time
Remember that for this semester, the assignments (including this one) are optional, not required.
Check the schedule for the due date. The class's Late Policy applies here. Remember that you should not modify turned in work after the due time has passed, so that when we grade it, it's not time-stamped late.
- Folders and files have the required names and are uploaded to the proper location.
- Your files have comments at the top and as necessary interspersed in the code.
- You have not modified the original HTML pages.
- Correctness and quality of the js and CSS code.